How to check if an object has stopped moving in unity
-
by Christian Engvall
- June 6, 2015
- 0
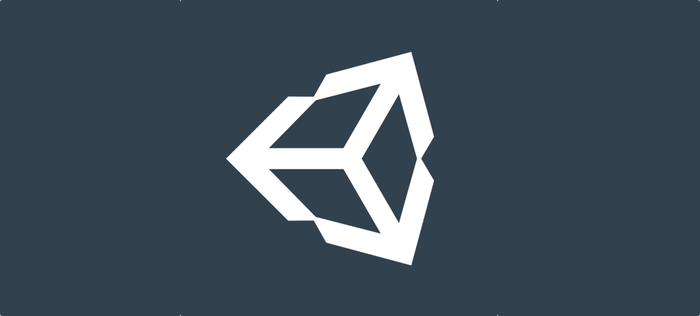
There were a few suggestions on how to check if a gameobject has stopped moving in unity. Some of them were scripts that did the trick. However the solutions that worked best was to check if the objects rigidbody2d isSleeping();
This Technique is used in Friendsheep.
using UnityEngine;
using System.Collections;
public class StuckSheepCheck : MonoBehaviour {
public GameObject Sheep;
public GameObject SheepTwo;
private Rigidbody2D SheepRigidbody2D;
private Rigidbody2D SheepTwoRigidbody2D;
void Start() {
this.SheepRigidbody2D = Sheep.GetComponent();
this.SheepTwoRigidbody2D = SheepTwo.GetComponent();
}
void FixedUpdate () {
this.CheckIfSheepIsStuck();
}
private void CheckIfSheepIsStuck() {
if(this.SheepRigidbody2D.IsSleeping() && this.SheepTwoRigidbody2D.IsSleeping()) {
SheepGameEnder.Instance.EndGame();
}
}
}
in Start() GetComponent<Rigidbody2D>() is used to get the rigidbodies from the gameObject(s) that will be checked if they are moving or not.
in fixedUpdate() a call to CheckIfSheepIsStuck() is made.
That method uses the rigidbodies that was collected in the startmethod to check if the gameobjects have stopped moving by calling the IsSleeping() method on the rigidbody(line 21). If both objects have stopped moving in this case the game ends. line 22 is not a unity-api method so replace that line with your own logic.